New since 2020/04: setPosition function for tabs. By default, the tabs will be inserted above remaining fields. Now you can decide if you want to display tabs above or below remainig fields. See chapter "SetPosition" at the bottom of this page.
Create custom tabs and move fields inside:
Table of Contents
Syntax
// file: hooks/TABLENAME-dv.js // get an instance of AppGiniDetailView var dv = AppGiniHelper.DV; // create tab var tab = dv.addTab( "name", "title", "icon" ); // add single field: tab.add( "field1" ); // add multiple fields (array of fieldnames): tab.add( ["field1", "field2", "field3"] ); // add a complete layout row: // create a layout-row (widths: 8/12 + 4/12), add some fields to columns 1 and 2 var row = dv.addLayout([8, 4]) .add(1, ["title", "date", "time"]) .add(2, ["status"]); // now add insert the whole row into the tab-panel tab.add( row );
Examples
New Tab with fields
Create a tab named "meta-tab" with title "Metadata" and Glyphicon "glyphicon-cog":
// file: hooks/events-dv.js var dv = AppGiniHelper.DV; var tab = dv.addTab( "meta-tab", "Metadata", "cog" ); .add("created_by") .add(["modified_by", "modified_on"]);

New Tab with multi-column layout
Step 1: Create a multi-column layout row with 8/12 + 4/12 and add some fields
// file: hooks/events-dv.js var dv = AppGiniHelper.DV; var row_2 = dv.addLayout([8, 4]) .add(1, ["title", "date", "time"]) .add(2, ["status"]);

Step 2: Move that row into a newly created tab
// ... dv.addTab("appointment-tab", "Appointment", "calendar") .add(row_2);

Complete code
// file: hooks/events-dv.js var dv = AppGiniHelper.DV; dv.compact(); var row_1 = dv.addLayout([12]) .add(1, ["name_patient"]) .sizeLabels(2); var row_2 = dv.addLayout([8, 4]) .add(1, ["title", "date", "time"]) .add(2, ["status"]); var row_3 = new AppGiniLayout([12]) .add(1, ["prescription", "diagnosis", "comments"]) .sizeLabels(2); dv.addTab("appointment-tab", "Appointment", "calendar") .add(row_1) .add(row_2); dv.addTab("details-tab", "Details", "search") .add(row_3); dv.addTab("meta-tab", "Metadata", "cog") .add(["created_by", "modified_by", "modified_on"]);
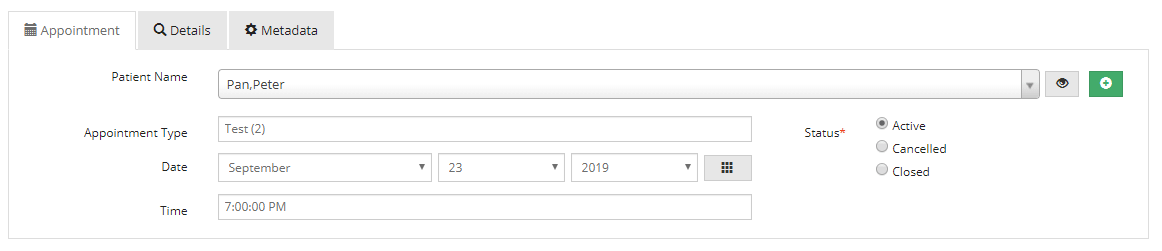
Another example from patients-dv.js
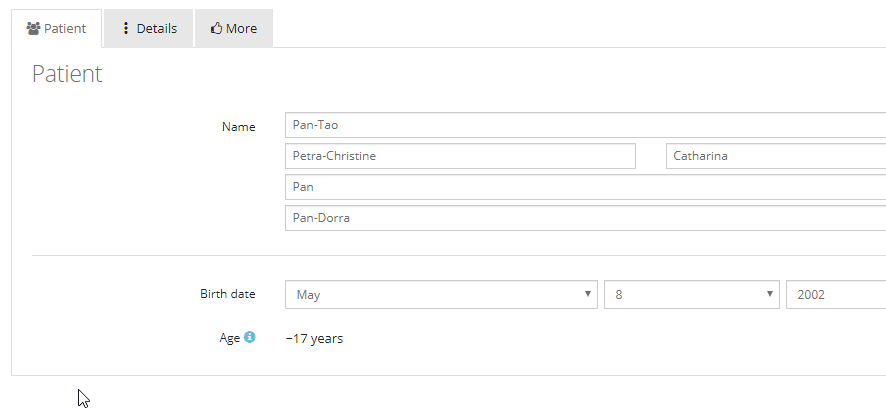
SetPosition
Be default, tabs will be inserted above existing fields. If you do not add all fields to tabs, there will be fields remaining below the tabs. With the new setPosition function you can switch positions.
Default
var dv = AppGiniHelper.DV; dv.addTab("tabGeneral", "Allgemein", "pencil", ["type_id","name", "description"]); dv.addTab("tabCalculation", "Abrechnung", "euro", ["rate", "type_rate"]);
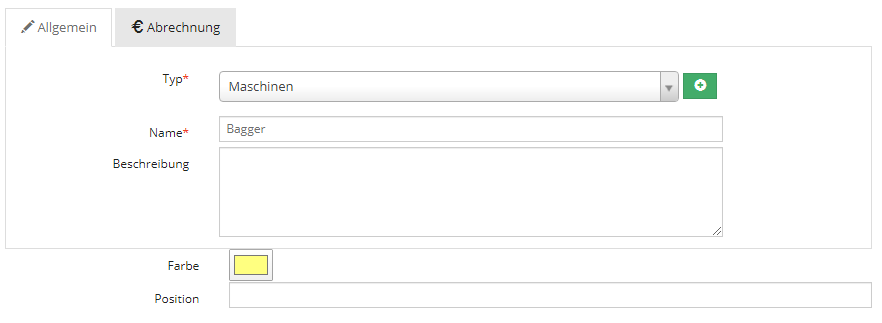
Move tabs below remaining fields
dv.getTabs().setPosition(TabPosition.Bottom);
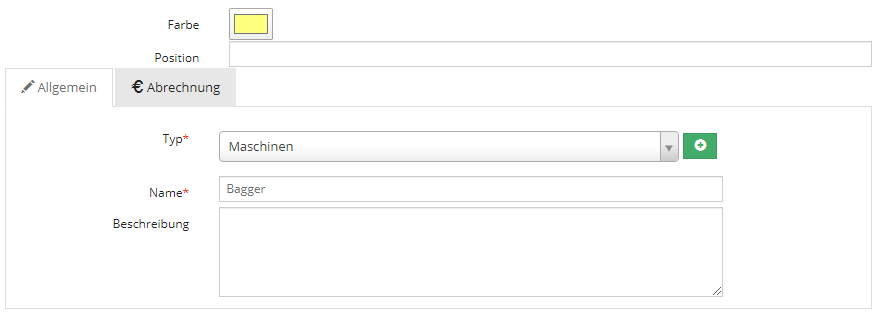
Syntax
var position = TabPosition.Bottom; // or // var position = TabPosition.Top; AppGiniHelper.DV.getTabs().setPosition( position );
Tip: Need some extra space?
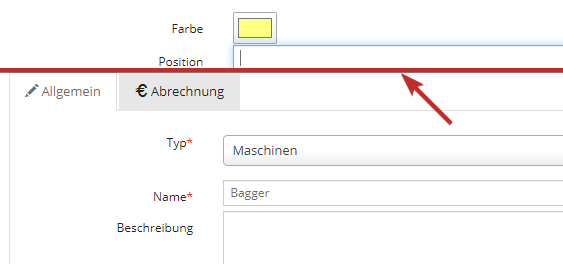
(1) Insert an empty paragraph
dv.getTabs().setPosition(TabPosition.Bottom); dv.getField("position").insertBelow().p();
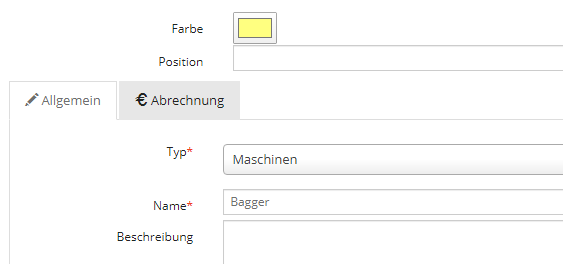
or (2) Insert a divider line
dv.getTabs().setPosition(TabPosition.Bottom); dv.getField("position").insertBelow().hr();
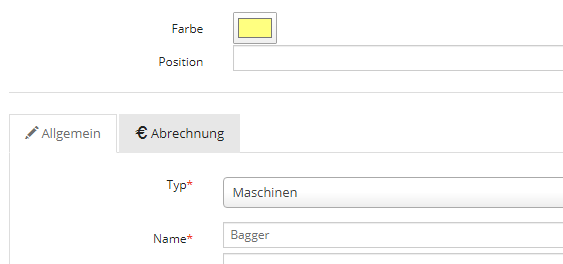
Do you like it?
We can only get better if you give us constructive suggestions for improvement. Just voting "No" without giving reasons or suggestions is not helpful and cannot lead to changes.
If you have been searching for a completely different solution than the subject says, this article can not be and will not be helpful for you. In these cases you should consider not to vote.
This is website feedback, only. This voting is not a support form nor ticket system.