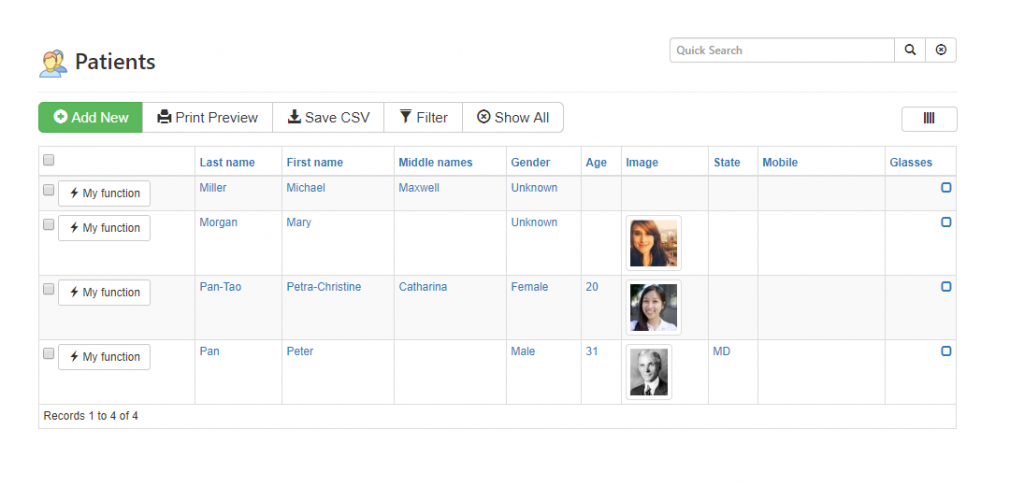
Note the custom buttons in first column of every row.
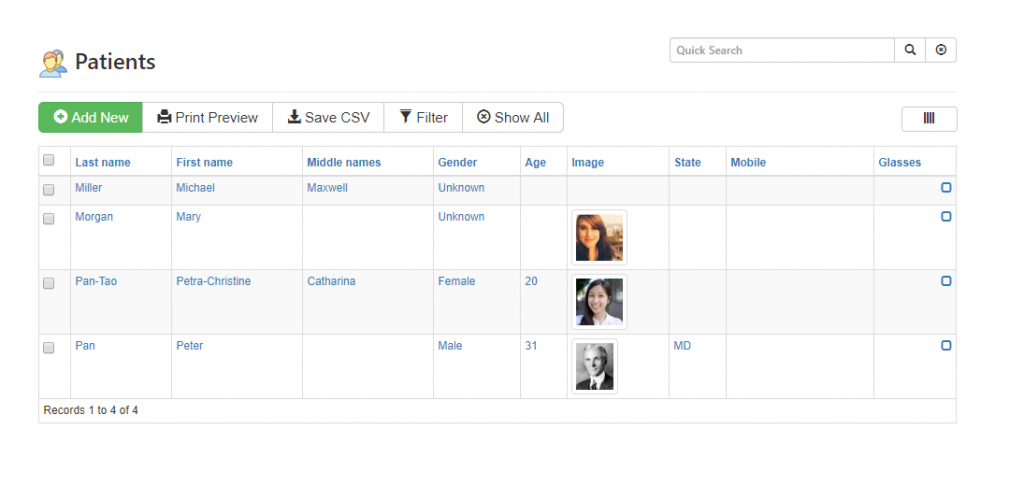
// file: hooks/patients-tv.js jQuery(function () { var tv = AppGiniHelper.TV; tv.addButton(function (id, data) { console.log(id); console.log(data); alert("User clicked #" + id); }, "flash", "My function"); // "flash" is the name of the glyphicon // "My function" is the button text });
Tipp
Instead of executing Javascript function you can also add links. See here.
Syntax
AppGiniHelper.TV.addButton(callback, icon, text, variation, onBeforeRender);
Parameters
callback
- Before 2021/07
function (id) { /* your code here */ }
1st
parameter: primary key of that row - Since 2021/07
function (id, data) { /* your code here */ }
1st
parameter: primary key of that row2nd
parameter: data of that row (as strings)
- Before 2021/07
icon
optional, string, eg. "cog", see also Glypgiconstext
optional, string, Button Text, eg. "Open"variation
optional, Variation, e.g. Variation.dangeronBeforeRender
new since 2021/07
A function which will be executed before rendering a button (per row). This function allows you to customize the button per row. See example at the bottom of this page
Please note
The .addLink()
method (see here) has a prompt parameter which, if set, automatically displays a confirmation prompt.
This .addButton()
method does not have a prompt parameter. But you can show the alert or confirmation prompt in your code - if required.
- Add button named "My function"
with icon "glyphicon-flash" - On click
- get last name
- get first name
- concatenate a text
- show alert
// file: hooks/patients-tv.js jQuery(function () { var tv = AppGiniHelper.TV; tv.addButton(function (id) { var last_name = tv.getValue(id, "last_name"); var first_name = tv.getValue(id, "first_name"); var text = "Clicked item #" + id + ": " + last_name + ", " + first_name; customAlert(text); }, "flash", "My function"); });
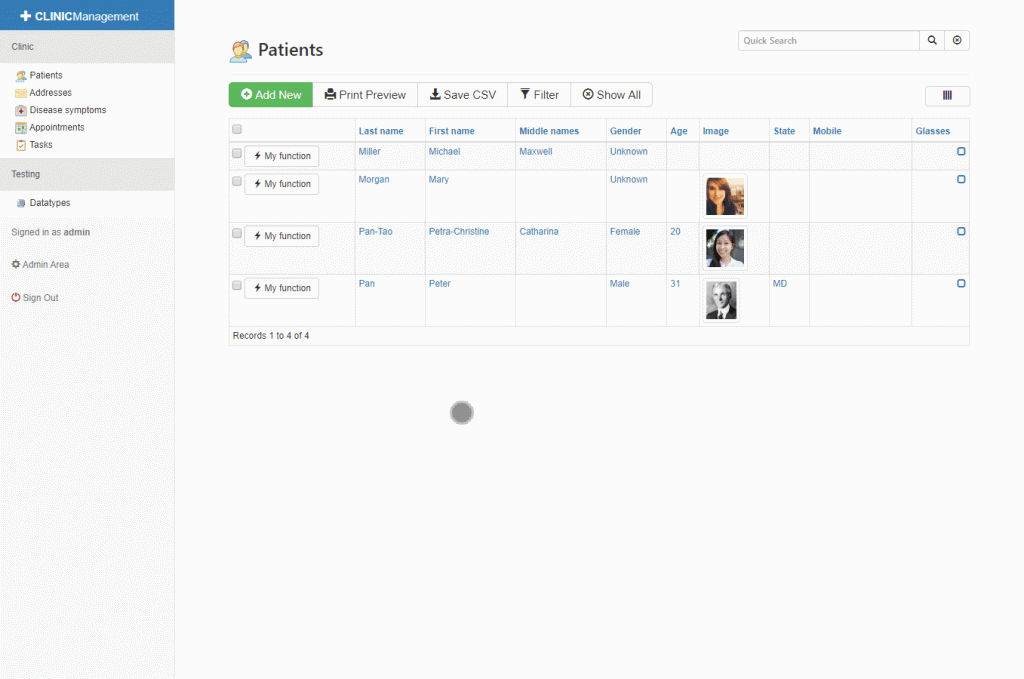
See also
Example for onBeforeRender
parameter
Using a callback function as 5th parameter you can modify the button per row. You can even skip rendering a button if this is not required in specific rows.
AppGiniHelper.TV.addButton( function (id, data) { } , "shopping-cart" , "Kaufoptionen" , Variation.default , function (id, data) { // your code here console.log(id); console.log(data); data.text = "text"; // custom text for this row data.icon = "cog"; // custom icon for this row data.variation = Variation.primary; // custom variation for this row data.skip = false; // when skip=true the button will NOT be rendered } );
Tip
I recommend console.log(data)
to see all available options
Do you like it?
We can only get better if you give us constructive suggestions for improvement. Just voting "No" without giving reasons or suggestions is not helpful and cannot lead to changes.
If you have been searching for a completely different solution than the subject says, this article can not be and will not be helpful for you. In these cases you should consider not to vote.
This is website feedback, only. This voting is not a support form nor ticket system.