Please note there has been an update on 2020/03/03 in toggleAlertAfterLoad()- function. For compatibility reasons there is one additional line of code. If the first version does not work for you, please replace that function by the new version which has already been updated on this page.
In this tutorial I am going to show how to toggle a custom alert on checking/unchecking a checkbox in AppGini detail view forms.
This is how it will look like in the end:

I am using out AppGini Helper Javascript Library which you can order here and also some undocumented BETA features.
Table of Contents
Prerequisites: Model and prepare a checkbox field
Ensure you have a checkbox field in your table. I am usually using integer datatype. For this example I have named the field int_checkbox
and configured is as integer
with [X] Checkbox
-option:
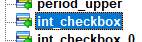
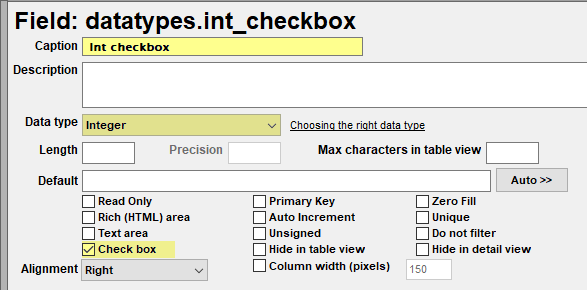
Generate your application and check if the new field renders correctly in your detail view form:
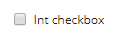
Step 1: Create a custom alert (and initially hide it)
Because we want to change the detail view, we have to make the changes in our TABLENAME-dv.js
hook file. In this example, I am editing hooks/datatypes-dv.js
, as "datatypes" is the name of my table
I am using the InsertBelow()-function described here for dynamically creating an alert below this field.
var fieldname = "int_checkbox"; var alert_message = "This is my custom message for the warning"; var alert_variation = Variation.Warning; new AppGiniField(fieldname) .insertAfter().alert(alert_message, alert_variation, "alert-on-check hidden");
Please note that I am adding the css class hidden
which automatically hides the newly created alert at the beginning. We are going to control this later depending on the current state of our checkbox. For now: hide the alert on page load.
Step 2: Listen for checkbox-changes
Next we have to detect the clicks on our checkbox field. We can use the (yet undocumented) BETA function onChange
which will be fired at any time the user changes our field.
Please note that this is BETA. It should work on most of the common datatypes but not on special types like Google Map or YouTube Video.
new AppGiniField(fieldname) .onChange(toggleAlert); function toggleAlert(value) { // this will be called on change }
The full code up to this point:
var fieldname = "int_checkbox"; var alert_message = "This is my custom message for the warning"; var alert_variation = Variation.Warning; // initialize onChange handler for field, then insert an alert below the field new AppGiniField(fieldname) .onChange(toggleAlert) .insertAfter().alert(alert_message, alert_variation, "alert-on-check hidden"); function toggleAlert(value) { // ... console.log(value); }
Save the file and reload the page in the browser.
Important note
Always clear the browser's cache on reload after you have changed javascript code or stylesheets. By doing this you can force the browser to load the latest versions of your code and not an outdated version from the browser's cache. See the troubleshooting guide for more information and keyboard shortcuts.
Click the checkbox and watch the console output of your browser's development tools. On every click it should show the value of the checkbox:
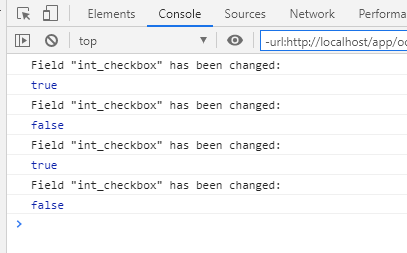
Step 3: Toggle visibility of our custom alert depending on checkbox state
Now that we can listen to changes, we can show or hide the custom alert. Therefore we modify the toggleAlert()
function.
function toggleAlert(visible = true, className = "alert-on-check") { var alert = jQuery("." + className); if (!alert.length) console.error("Alert not found: ." + className); else { if (visible) { alert.removeClass("hidden"); } else { alert.addClass("hidden"); } } }
Complete code at this point:
var fieldname = "int_checkbox"; var alert_message = "This is my custom message for the warning"; var alert_variation = Variation.Warning; // initialize onChange handler for field, then insert an alert below the field new AppGiniField(fieldname) .onChange(toggleAlert) .insertAfter().alert(alert_message, alert_variation, "alert-on-check hidden"); function toggleAlert(visible = true, className = "alert-on-check") { var alert = jQuery("." + className); if (!alert.length) console.error("Alert not found: ." + className); else { if (visible) { alert.removeClass("hidden"); } else { alert.addClass("hidden"); } } }
Result at this point:

Everything should work pretty well at this point.
Don't worry if this does not work, yet. As mentioned at the top of this page, there was a problem with backward compatibility. Please continue with the next step. There is a bugfix below and it should work in the end.
Now, the only thing left is: What if the checkbox is checked on page load. I'd like to see the alert immediately. But as written above, I am initially hiding the alert. So we now need to detect the current state on page load and then conditionally show the alert.
Step 4: Initially show or hide our custom alert depending on initial checkbox state
There is only one piece left. We need to detect the initial checkbox value immediately after the page has loaded. If the checkbox is checked, we have to show the custom alert.
var dv = AppGiniHelper.DV; // as soon as the pages has loaded completely, call a function dv.ready(toggleAlertAfterLoad); // toggle visibility of our alert depending on the curren checkbox state function toggleAlertAfterLoad() { // UPDATE 2020/03/03 // for backward compatibility please insert the following line $j("#" + fieldname).closest(".checkbox").next().addClass("alert-on-check"); var is_checked = $j("#" + fieldname).attr("checked") !== undefined; toggleAlert(is_checked); }
The full code up to this point:
var dv = AppGiniHelper.DV; var fieldname = "int_checkbox"; var alert_message = "This is my custom message for the warning"; var alert_variation = Variation.Warning; // initialize onChange handler for field, then insert an alert below the field new AppGiniField(fieldname) .onChange(toggleAlert) .insertAfter().alert(alert_message, alert_variation, "alert-on-check hidden"); // initially toggle the alert depending on the curren checkstate dv.ready(toggleAlertAfterLoad); function toggleAlertAfterLoad() { $j("#" + fieldname).closest(".checkbox").next().addClass("alert-on-check"); var is_checked = $j("#" + fieldname).attr("checked") !== undefined; toggleAlert(is_checked); } function toggleAlert(visible = true, className = "alert-on-check") { var alert = jQuery("." + className); if (!alert.length) console.error("Alert not found: ." + className); else { if (visible) { alert.removeClass("hidden"); } else { alert.addClass("hidden"); } } }
That's it. If the checkbox was checked on last save, the alert should be visible now on page load.
Please share this post if it works for you!
Update 2020/03/03: Modified function
function toggleAlertAfterLoad() { $j("#" + fieldname).closest(".checkbox").next().addClass("alert-on-check"); var is_checked = $j("#" + fieldname).attr("checked") !== undefined; toggleAlert(is_checked); }