For the project I have already mentioned here and here, a barcode scanner is used for various tasks. If the input cursor is not in a search field, but in an input field, then the value of the field is overwritten by the scanned value or appended to the existing value. Sometimes this is on purpose, for example when creating a new record and assigning a Barcode to it. Most of the times overwriting an existing value by scanning a Barcode would cause data loss on save. To avoid this I have implemented a write protection for <input />
-fields.
When scanning a barcode with a standard barcode scanner, the recognized value is treated as a keyboard entry. If the cursor is positioned in an input field, the recognized barcode is written there. If a user does not pay attention to which field the input cursor is currently in when scanning, this could cause data loss. This is especially the case when the user is not sitting directly in front of the monitor, but is using a wireless barcode scanner several meters away, not watching the screen all the time.
When I enter a Detail View, AppGini places the cursor on a defined or on the first available input field according to certain rules. This is usually very useful. In my case it would look like this:
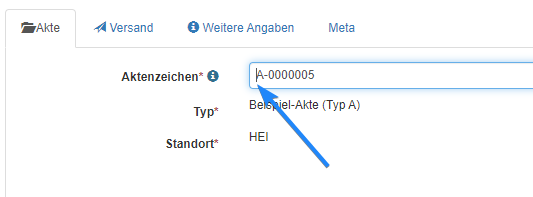
If the user were to scan a barcode now, the following would happen:
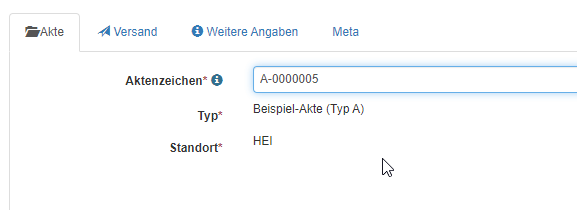
If the user is inattentive at this moment, he might create quite undesirable results or states when saving the dataset.
To avoid this, I have written a Javascript function that allows me to lock the field:
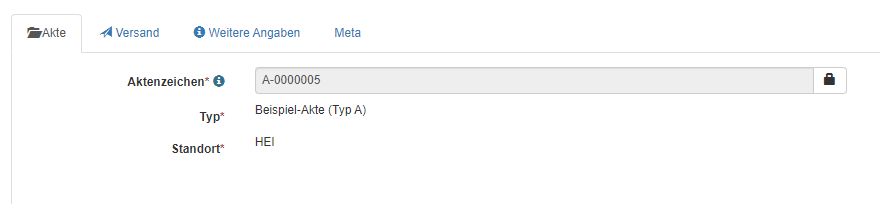
Note that the field is readonly now and got an additional lock/unlock button. When scanning, this value will not overwritten any longer.
If the user really intends to change the value, he/she must first unlock the field:
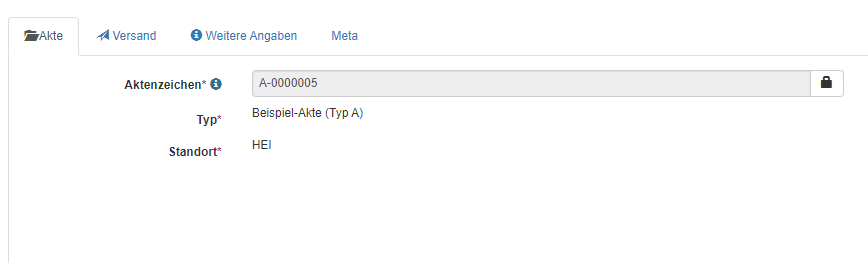
This looks like a little more work for the user when editing. In practice, however, this is not the case, because the value is usually not changed after the first creation of a new record. In addition I note that the lock is NOT set in insert-mode.
Limitations
The function is available to me for simple <input />
-fields, only.
For lookups and other complex field-types which require a mouse action, not just keyboard input, it doesn't make sense in my case.
Javascript Code
The code for the detail view looks like this right now:
// file: hooks/items-dv.js var dv = AppGiniHelper.dv; if (dv.isInsert()) dv.getField("reference").lock(false); else dv.getField("reference").lock();
The lock function itself modifies the <input/>
-field. The boolean argument false
| true
(default) controls the initial state.
I'm using AppGini Helper's dv.isInsert()
-function to determine if we are in Insert-mode or not. In both cases (Insert-Mode or Edit-Mode) I initialize the locking by calling the lock()
-function on the field. In Insert-Mode I pass false
, which means: initialize locking on that field, but don't actually lock it. In Edit-Mode I don't pass an argument. The default value is true
. This means: initialize locking on the field and lock it now.
The code above should be readable and understandable especially for beginners. In practice the code looks like the following, which, actually, is the same, but shorter:
dv.getField("reference").lock(!dv.isInsert());
Summary
For me it works perfectly now. If this feature proves successful over time, I plan to include it in the next version of the AppGini Helper Javascript Library.