Adding buttons and adding links to a table view has been described in the docs. By using the %ID%
placeholer you can use the record's primary key as parameter for links. For example for opening the record in detail view. In this article we are going to use the %ID%
parameter for adding a sub-item (child record / detail record) to the row.
The Online Clinical Management System (OCMS), which I'm using in many examples, contains patients and appointments. Appointments are stored in a table named events
. And the column name_patient
has been configured as lookup field refering patients
table.
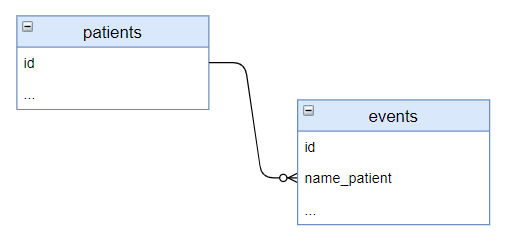
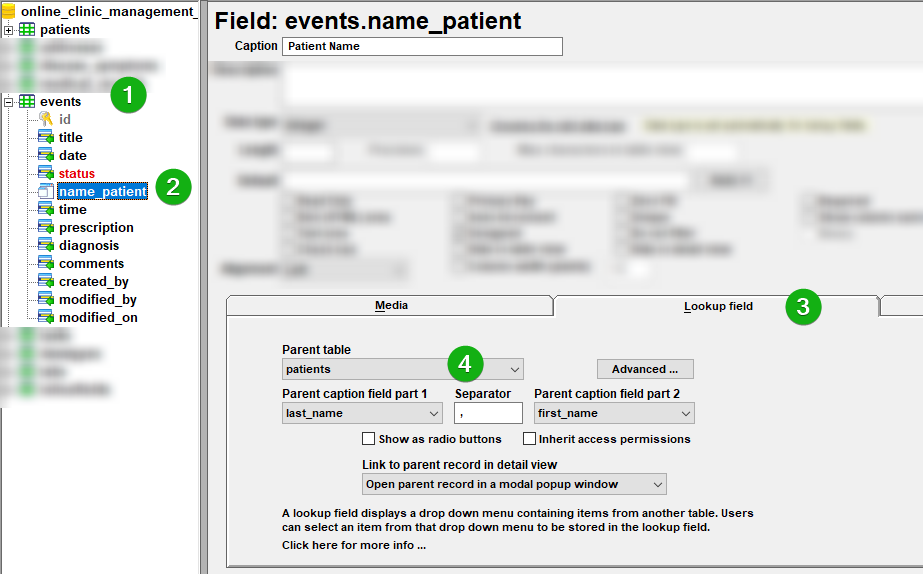
Step 1: Button for opening details
Let's get started with adding a link (button) to patients
table view for opening up one patient detail view record.
The URL-pattern (address) for a detail view record isTABLENAME_view.php?SelectedID=THE_RECORDS_PRIMARY_KEY
.
Example:
The URL for opening the detail view of patients
table for record #1
patients_view.php?SelectedID=1
.
If we know the URL, we can add a button to table view.
Create (or edit) a hooks file file table view named hooks/TABLENAME-tv.js
(replace TABLENAME by the name of your specific table).
// file: hooks/patients-tv.js jQuery(function () { var tv = AppGiniHelper.TV; // additional buttons for table view // add "open" button var tablename = AppGini.currentTableName(); var url_open = tablename + "_view.php?SelectedID=%ID%"; tv.addLink(url_open, "pencil", "", Variation.primary); });
The screenshot show the result: The blue "pencil" buttons have been added to table view. A click opens up the detail view of that record.
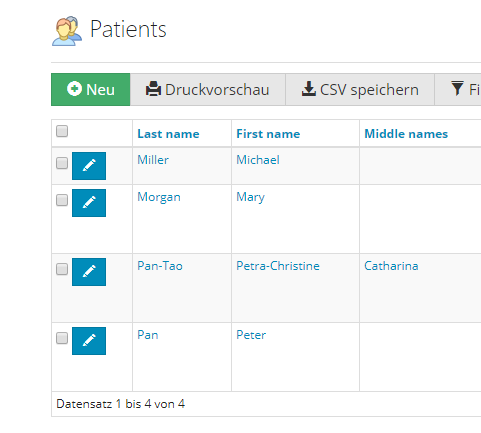
Step 2: Create button for adding an appointment
Finding the URL for adding a subitem is a bit difficult. But don't worry: I will guide you. By using special parameters we can tell AppGini to open up the "Add new record" view for a table. The required parameter is addNew_x
.
URL for adding new record to events
table:
events_view.php?addNew_x=1
Note the ?
character which separates the page (events_view.php
) from one or more parameters.
Additionally we can pass default values to the view by using filterer_COLUMNNAME
parameters.
In this case we need to pass the patient's primary key (value of patients
.id
) as default value for the new event's foreign key (column events
.patient_name
).
URL for adding a new event
record as subitem refering patient
#1:
events_view.php?addNew_x=1&filterer_name_patient=1
Note the &
character for concatenating additional parameters. Also note that filterer_name_patient
matches the syntax of filterer_COLUMNNAME
, because our foreign key column name is name_patient
.
You might wonder: The primary key of the master record (patient
) changes from row to row. It is not a static value like 1
as we have seen in the example above. So, the question is: how can we pass the primary key for each individual patient
-record?
We can use the placeholder %ID%
to pass in the primary key of our patients table row by row.
events_view.php?addNew_x=1&filterer_name_patient=%ID%
Now we have our URL. So we can add a new button to our table view. In the following code I'm using a couple of variables to make the code easier to read, understand and copy/paste for you.
var details_table_name = "events"; var details_fk_column_name = "name_patient"; var url_add_detail = details_table_name + "_view.php?addNew_x=1&filterer_" + details_fk_column_name + "=%ID%"; tv.addLink(url_add_detail, "calendar", "+");
Full source code:
jQuery(function () { var tv = AppGiniHelper.TV; // additional buttons for table view // add "open" button var tablename = AppGini.currentTableName(); var url_open = tablename + "_view.php?SelectedID=%ID%"; tv.addLink(url_open, "pencil", "", Variation.primary); // add "add appointment" button var details_table_name = "events"; var details_fk_column_name = "name_patient"; var url_add_detail = details_table_name + "_view.php?addNew_x=1&filterer_" + details_fk_column_name + "=%ID%"; tv.addLink(url_add_detail, "calendar", "+"); });
Result
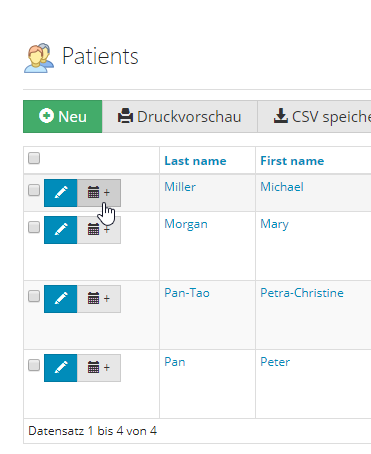
On click, our button opens up the "Add-New Event" form with a predefined relation to this patient (Miller, Michael in this case):
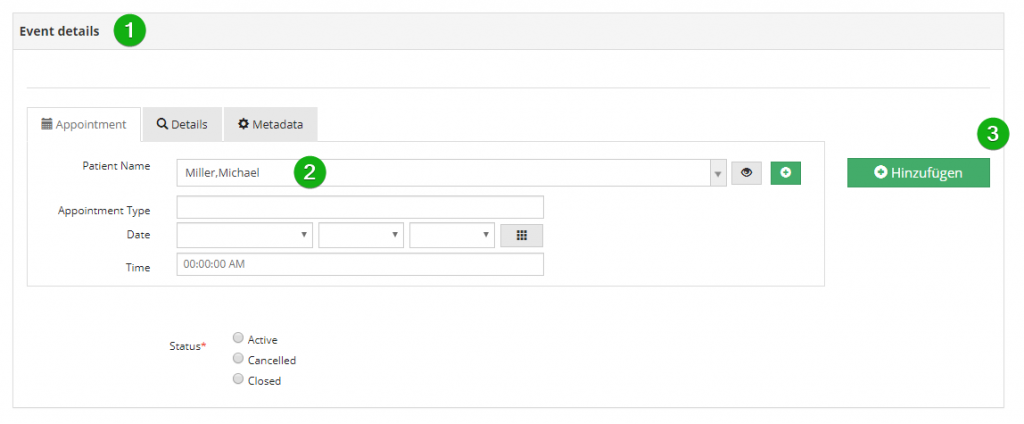
As you can see, we are in "add new" mode of events
-table. The default value for the (parent) patient has been set correctly. This gives many, many options for your projects.
Full short code with one additional button for listing all appointments of a patient:
// file: hooks/patients-tv.js jQuery(function () { var tv = AppGiniHelper.TV; // additional buttons for table view // add "open" button var tablename = AppGini.currentTableName(); var url_open = tablename + "_view.php?SelectedID=%ID%"; tv.addLink(url_open, "pencil", "", Variation.primary); // add "list all appointsments of patient" button var url_appointments = "events_view.php?filterer_name_patient=%ID%"; tv.addLink(url_appointments, "calendar"); // add "add appointment" button var url_add_detail = "events_view.php?addNew_x=1&filterer_name_patient=%ID%"; tv.addLink(url_add_detail, "calendar", "+"); });
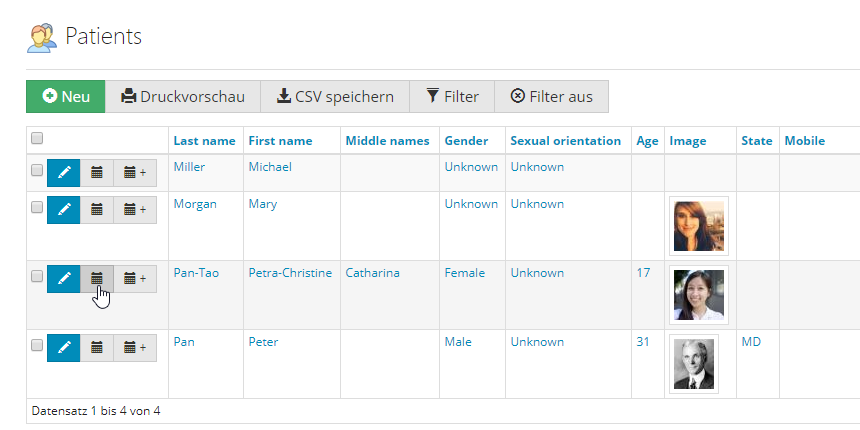
Hope you like it. If you like it, please follow or like us on twitter and share this piece of information.