In Part 1 we have seen how to change a value on inserting a new record. Now we are going to change multiple values at once using the same technique.
Table of Contents
Step 1 and Step 2
Pease follow Step1 and Step 2 from Part 1 of this tutorial.
Step 3: Define fieldnames and values
A good way for defining multiple fieldname+value tuples in PHP is by using associative arrays:
$my_array = []; $my_array["key1"] = "value1"; $my_array["key2"] = "value2"; // ...
A shorter variant:
$my_array = [ "key1" => "value1", "key2" => "value2" // ... ];
Please note the assignment operator =>
between key and value! From my own experience, sometimes you are searching for a bug for minutes and it's just that you have been using equal-sign =
instead of assignment =>
operator.
I am using an associative array for defining my tuples of fieldname
and default value
like this:
Once again, this is an example and not a real world scenario.
$init_data = [ "label" => $memberInfo["custom"][0], "last_name" => "Io", "first_name" => "Europa", "middle_names" => "Ganymed", "memberID" => $memberInfo["username"] ];
Step 4: Change the values
For multiple values I'm using the same technique as shown in Part 1, Step 4. But with multiple values it is a bit more complicated. I have put the code into a single PHP line which you can copy and paste:
$html .= '<script>' . implode("\r\n", array_map(function($fieldname, $value) { return "jQuery('#{$fieldname}').val('{$value}');"; }, array_keys($init_data), $init_data )) . '</script>';
Complete Code
function contacts_dv($selectedID, $memberInfo, &$html, &$args) { // multiple fields if (!$selectedID) { $init_data = [ "label" => $memberInfo["custom"][0], "last_name" => "Io", "first_name" => "Europa", "middle_names" => "Ganymed", "memberID" => $memberInfo["username"] ]; $html .= '<script>' . implode("\r\n", array_map(function($fieldname, $value) { return "jQuery('#{$fieldname}').val('{$value}');"; }, array_keys($init_data), $init_data )) . '</script>'; } }
Final Result
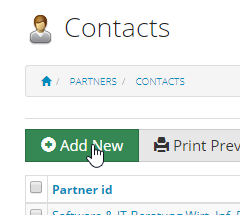
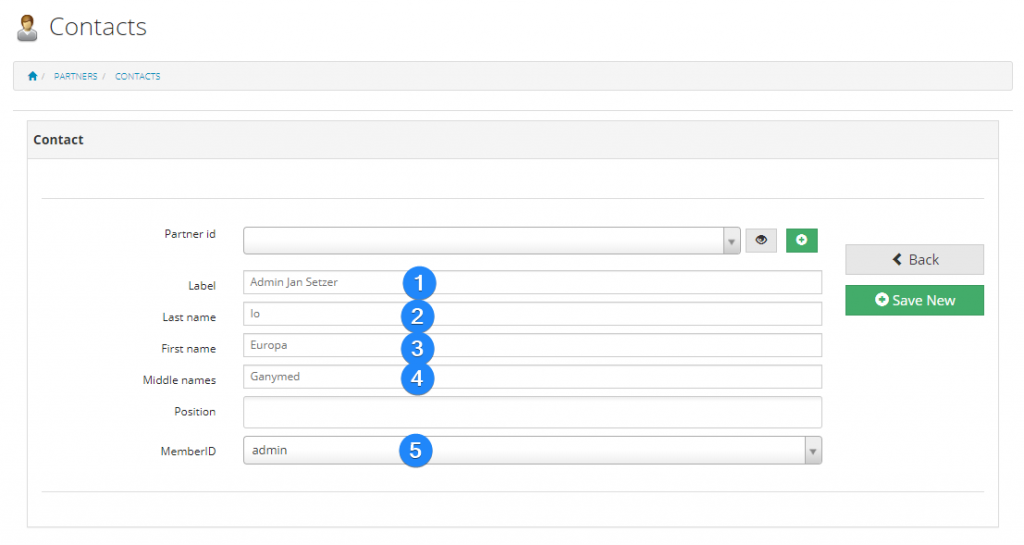
All fields have been populated with default values.
This should work for all fields which can be modified using jquery’s val("new value")
function.
Pingback: How to: Default-value hack for more flexibility (Part 1) | AppGini Helper