Today I am going to take you on a trip from a default, generated detail view to a UI-modified detail view using the latest version of our AppGini Helper Javascript Library (which is 2020/10/26). Follow me!
Table of Contents
Start
This is the default generated attachments
detail view form (DV) with a couple of fields for uploading PDF files:
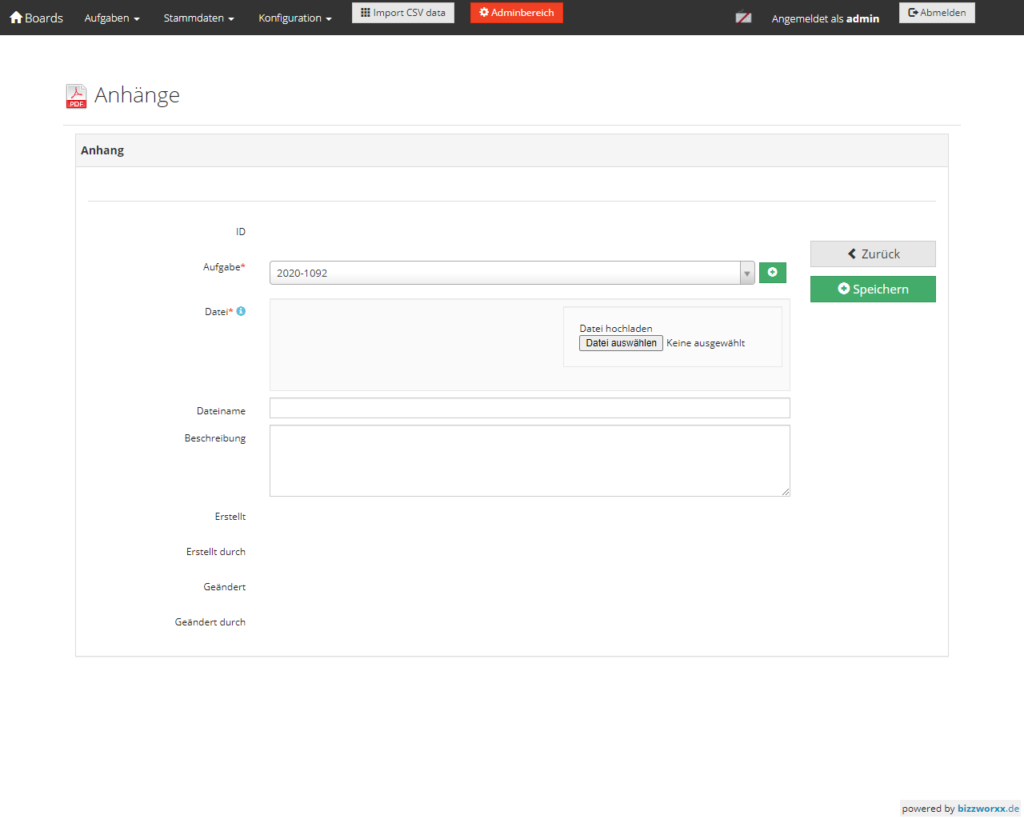
Schema
I'm using the following data model for this attachments
table:
- attachments
id
primary keytask_id
lookup tasks-tablefile
field for uploading pdf filesfilename
will be filled automatically on insertdescription
textare for descibing or tagging this filecreated_on
created_by
modified_on
modified_by
Create hooks file
Create a new file named attachments-dv.js in hooks directory
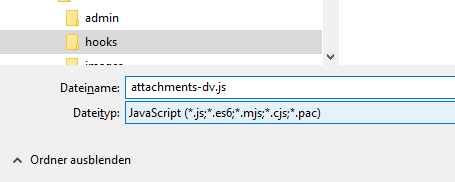
// file: hooks/attachments-dv.js
DV-Variable
Declare a variable which can be used for modifying the detail view
var dv = AppGiniHelper.DV;
Tabs
Add tab for main attachment fields
dv.addTab("tabAttachment", "Datei", "file", ["task_id", "file", "filename", "description"]);
Parameters: name, title, icon, array of fieldnames
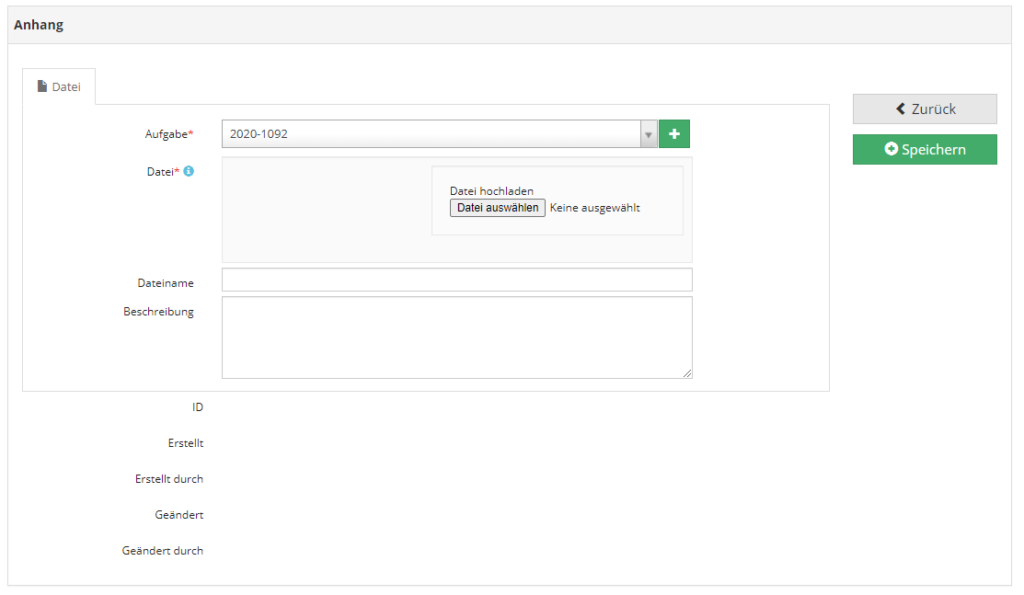
Add tab for meta data fields
dv.addTab("tabMeta", "Meta", null, ["id", "created_on", "created_by", "modified_on", "modified_by"]);
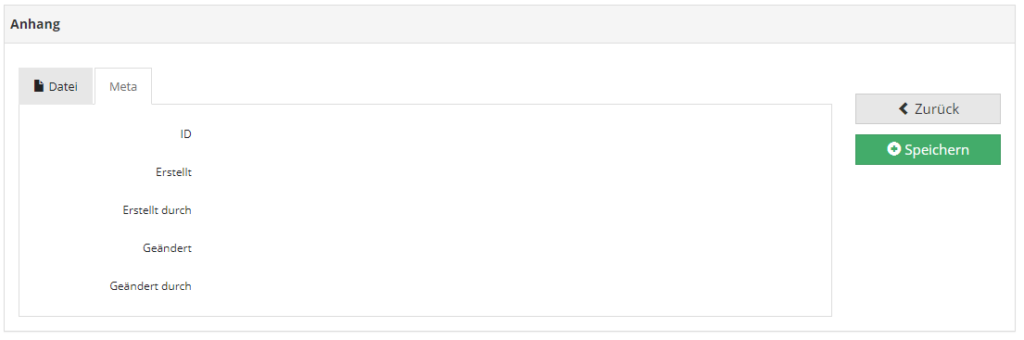
Field changes
Meta-Tab
Hide id-field
dv.getField("id").hide();
Main Tab
If we are in insert mode, hide filename
field. It will be populated automatically after insert (see description below).
So, for now there is no need to show an empty field in insert mode.
if (dv.isInsert()) { dv.getField("filename").hide(); }
Full Javascript code up to this point:
// file: hooks/attachments-dv.js var dv = AppGiniHelper.DV; dv.addTab("tabAttachment", "Datei", "file", ["task_id", "file", "filename", "description"]) dv.addTab("tabMeta", "Meta", null, ["id", "created_on", "created_by", "modified_on", "modified_by"]); dv.getField("id").hide(); if (dv.isInsert()) { dv.getField("filename").hide(); }
Result up to this point
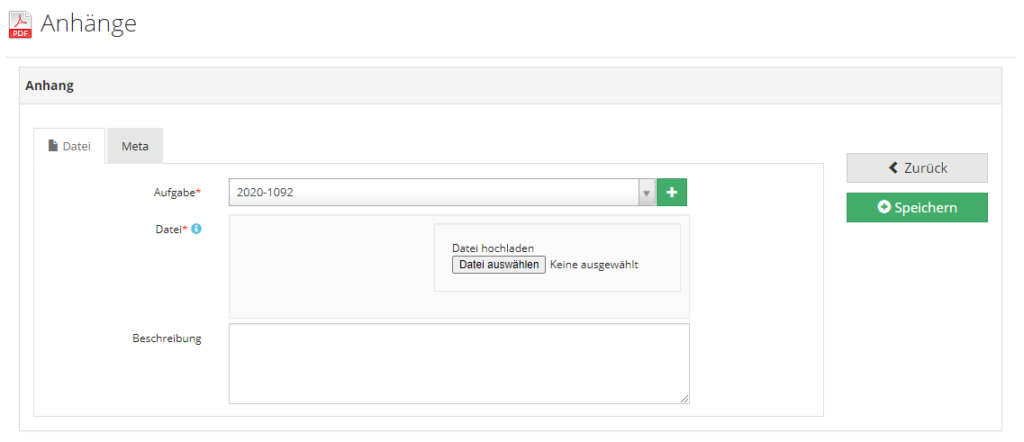
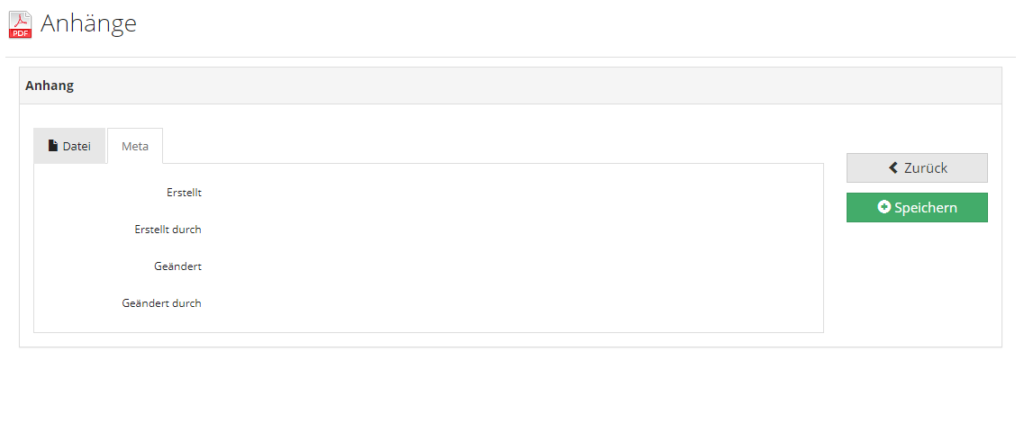
Auto-populate filename on upload
On upload, the file will be moved into images folder and renamed. But I'd like to keep the original filename and store it my filename
field. So I have to write PHP code in hooks/attachments.php
for populating that field on upload automatically.
// file: hooks/attachments.php // ... function attachments_before_insert(&$data, $memberInfo, &$args) { $data["filename"] = $_FILES["file"]["name"]; return TRUE; } // ...
Test upload
For testing purpses, select a file and insert the record
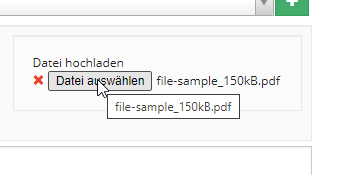
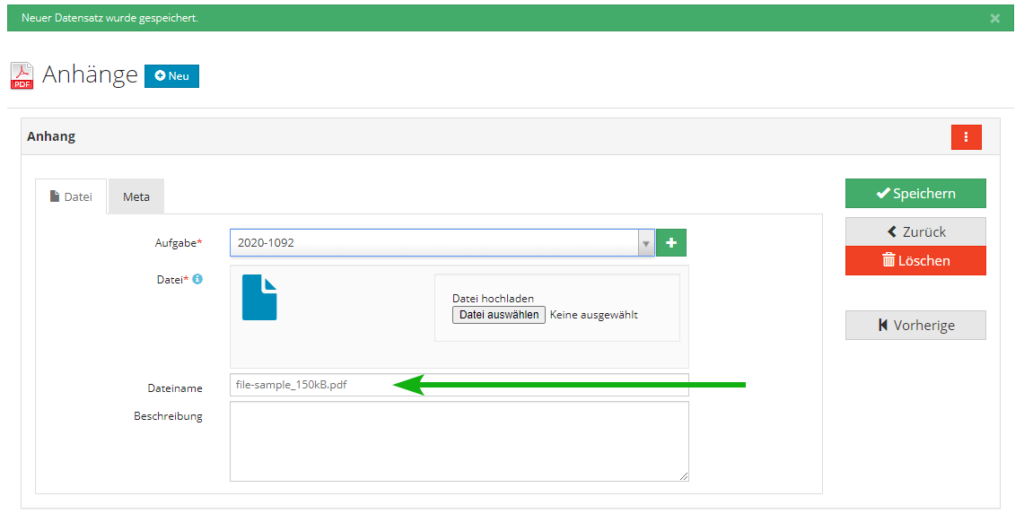
The file has been uploaded and the record has been inserted. As you can see (green arrow) the original filename has been stored, too. So my PHP code works.
Adjustments
Meta-Tab
For UI reasons I'd like to slightly modify the created-/modified- fields
dv.getFields(["created_on", "created_by"]).inline("Erstellt"); dv.getFields(["modified_on", "modified_by"]).inline("Zuletzt geändert");
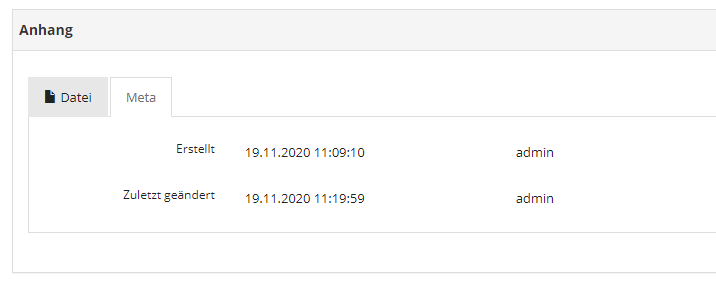
Main Tab
filename
field readonly in edit-mode
I don't want my users to change the original filename
. So, in a way I have to write protect this. In insert-mode we have hidden that field. In update mode (not insert-mode) I'd like to protect the filename field:
I replace the code fragment from above by the following:
if (dv.isInsert()) { dv.getField("filename").hide(); } else { dv.getField("filename").readonly(); }

After I have seen this result, for optical reasons I'd like to change the readonly (grey) field. There is another unsupported function which should work well for normal input fields. So I replace the .readonly call (see before) by a .toStatic() function call.
if (dv.isInsert()) { dv.getField("filename").hide(); } else { // dv.getField("filename").readonly(); dv.getField("filename").toStatic(); }

Please note that the .readonly()
-function and the .toStatic()
-function which I am using here are unsupported. They do work for many, many datatypes, subtypes and variations but I cannot guarantee for all situations. For normal input fields they should work just fine.
That's fine. Maybe it would be even better if I move filename to the meta-tab. So, this is the full code up to this point:
// file: hooks/attachments-dv.js var dv = AppGiniHelper.DV; dv.addTab("tabAttachment", "Datei", "file", ["task_id", "file", "description"]) dv.addTab("tabMeta", "Meta", null, ["id", "filename", "created_on", "created_by", "modified_on", "modified_by"]); dv.getField("id").hide(); if (dv.isInsert()) { dv.getField("filename").hide(); } else { // dv.getField("filename").readonly(); dv.getField("filename").toStatic(); }
Height of description textarea
I'd like to increase the height of the description
field a little bit. I could do this using CSS or JQuery but - you know me - I have a special and simple fuction call for that:
dv.getField("description").asTextarea().setRows(15);
Result
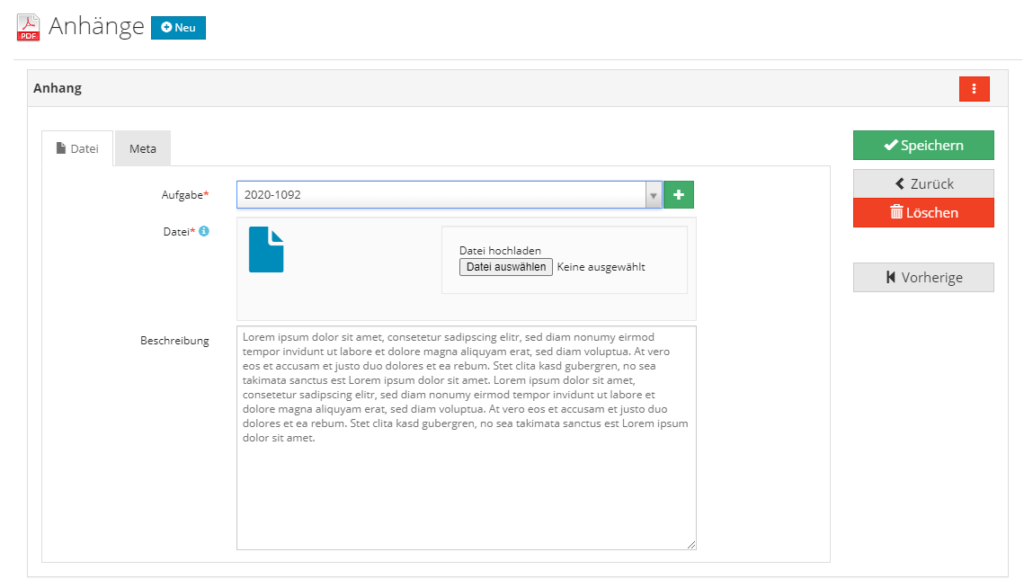
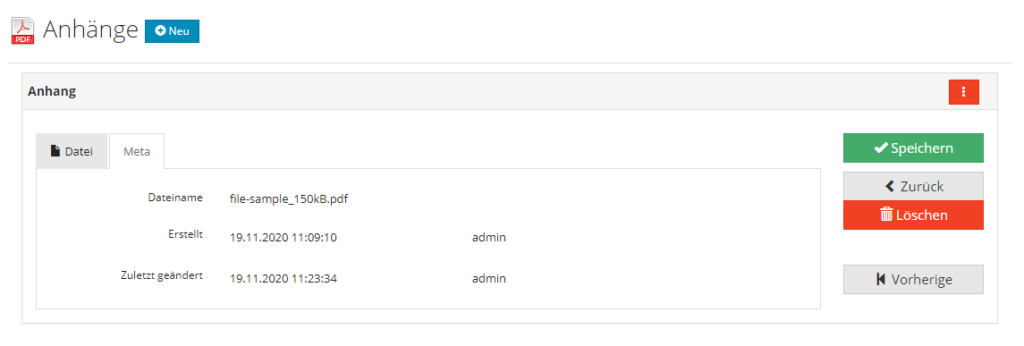
I'm not quite satisfied with the file-upload field/panel but that is another story. I hope you enjoyed this step-by-step example.
Keep coding, AppGineers!
Kind Regards,
Jan